Jetpack Compose: Rows
10th April 2022
Rows are one of the fundamental building blocks of compose. Think of them like a linear layout with a horizontal arrangement. Lets take a look at what makes a row:
@Composable
inline fun Row(
modifier: Modifier = Modifier,
horizontalArrangement: Arrangement.Horizontal = Arrangement.Start,
verticalAlignment: Alignment.Vertical = Alignment.Top,
content: @Composable RowScope.() -> Unit)
As rows are often used as a container for other content, we have access to a vertical alignment and horizontal arrangement properties which we can set.
There is also RowScope
which gives the row's children access to some more values which can be used to influence their layout and display.
Vertical alignment
Start, Center, Bottom
These values are pretty self-explanatory. They will stick the children vertically to the start center or end of the row
Row(verticalAlignment = Alignment.Top) {
Text(...)
Text(...)
}
Row(verticalAlignment = Alignment.CenterVertically) {
Text(...)
Text(...)
}
Row(verticalAlignment = Alignment.Bottom) {
Text(...)
Text(...)
}
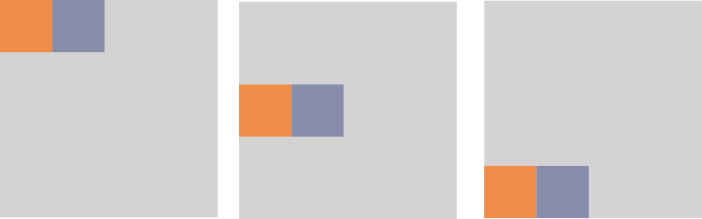
Horizontal arrangement
Start, Center, End
Again these values are pretty self-explanatory. They will stick the children horizontally to the start, center or end of the row
Row(horizontalArrangement = Arrangement.Start) {
Text(...)
Text(...)
}
Row(horizontalArrangement = Arrangement.Center) {
Text(...)
Text(...)
}
Row(horizontalArrangement = Arrangement.End) {
Text(...)
Text(...)
}
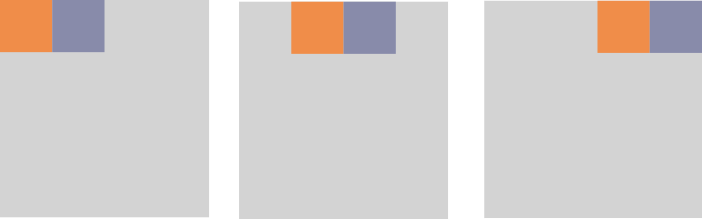
Space between
Space between will evenly distribute the children throughout the row without adding any additional spacing before the first of after the last child
Row(horizontalArrangement = Arrangement.SpaceBetween) {
Text(...)
Text(...)
}
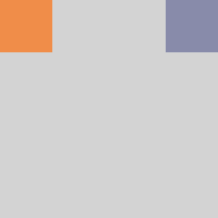
Space around
Shamelessly taken from the docs: Place children such that they are spaced evenly across the main axis, including free space before the first child and after the last child, but half the amount of space existing otherwise between two consecutive children. Visually: #1##2##3# for LTR and #3##2##1# for RTL
Row(horizontalArrangement = Arrangement.SpaceAround) {
Text(...)
Text(...)
}
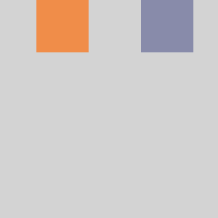
Space evenly
Place children such that they are spaced evenly across the main axis, including free space before the first child and after the last child. Visually: #1#2#3# for LTR and #3#2#1# for RTL
Row(horizontalArrangement = Arrangement.SpaceEvenly) {
Text(...)
Text(...)
}
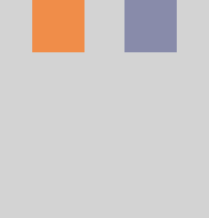
RowScope
Any composable created within our Row has access to its RowScope. This gives access to some useful modifiers that the children can make use of.
Align
The align modifier takes in an Alignment as seen above in the vertical examples. If a child has this set through a modifier it overrides any that is set on the parent. Allowing for a staggered set of views for example.
Row(...) {
Text(modifier = Modifier.align(Alignment.CenterVertically))
Text(...)
}
fun Modifier.align(alignment: Alignment.Vertical): Modifier
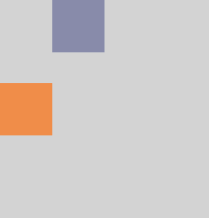
Weight
The weight modifier works much the same way as it does in HTML. It allows you to say that certain composables should take up greater portions of the screen than others.
For example of you have 2 Views - The first has a weight of 2 and the second has a weight of 1, the first View will take up 2/3 of the screen and leave 1/3 to the second view.
The fill property allows us to say that if a composable should take up 2/3 but its content only currently fills 1/3 then we will only draw it as 1/3 but the remaining space can't be taken by our other views.
Row(...) {
Text(modifier = Modifier.weight(1f))
Text(modifier = Modifier.weight(1f))
Text(modifier = Modifier.weight(1f))
}
Row(...) {
Text(modifier = Modifier.weight(1f)
Text(modifier = Modifier.weight(2f))
Text(modifier = Modifier.weight(1f))
}
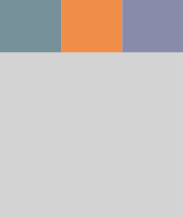
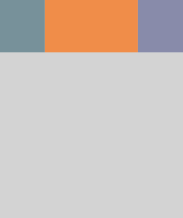